HTML id Attribute
Understanding the HTML id Attribute
In HTML, the id attribute serves as a unique identifier for any HTML element (example: divs, p, h1-h6). Using HTML Id you can target that specific element with CSS, JavaScript, or even links. Moreover, adhering to a clear html id naming convention ensures your code remains semantic.
Why Use an HTML id?
Firstly, an html id makes it easy to style an individual HTML element via a css id selector. Secondly, you can reference it in scripts using html id javascript methods like getElementById(). Finally, combining both anchor id html techniques allows user a smooth in-page navigation.
Examples of Using the HTML id Attribute
1. CSS HTML id Selector
You can target a single element using the CSS id selector by prefixing the id with # in your stylesheet, as shown in the example 1 below. In this code, the paragraph with id=”highlight” is styled with a yellow background and 10 pixels of padding. You can run this code by clicking on “Test my Code” button.
<!-- Example 3: HTML id selector in CSS -->
<style>
#highlight {
background-color: yellow;
padding: 10px;
}
</style>
<p id="highlight">This paragraph is highlighted.</p>
2. Anchor HTML id for In-Page Links
Example 2 shows how you can create an in-page link by setting an anchor’s link to id #features, which jumps directly to the element with id=”features”. Using id for anchor tag enables smooth navigation within a single HTML document.
<!-- Example 2: anchor html id -->
<a href="#features">Go to Features</a>
<br> <br> <br> <br> <br> <br> <br> <br> <br> <br> <br>
<br> <br> <br> <br> <br> <br> <br> <br> <br> <br> <br>
<!-- Later in the page -->
<h2 id="features">Key Features</h2>
<p>Details about our product’s key features.</p>
3. Accessing HTML id in JavaScript
The example below show how you can use HTML id with JavaScript. It uses document.getElementById(‘message’) to select the paragraph with id=”message” and updates its innerText when the button is clicked. It highlights how easily you can control specific elements by their unique HTML id in JavaScript.
<!-- Example 4: html id in JavaScript -->
<p id="message">Original message.</p>
<button onclick="document.getElementById('message').innerText = 'Updated via JavaScript!'">
Click Me
</button>
4. HTML Unique Identifier & Naming Convention
HTML IDs should be given meaningful names. The example below provides a clear html id naming convention by using a descriptive, prefixed identifier (id=”nav-main”) for the primary navigation element. Properly named IDs reinforces the importance of unique and meaningful IDs for enhance readability of the codes and to prevent conflicts in styling or scripting.
<!-- Example 5: Naming convention -->
<nav id="nav-main">
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
</ul>
</nav>
5. Combining HTML id with CSS and JavaScript
The example below combines a inline JavaScript event (onclick) that changes its background color on click, along with CSS html id css selector (#box) to define the box’s size, color, and cursor style. This approach demonstrates how styling and scripting can work hand-in-hand on a single element identified by its HTML id.
<!-- Example 6: Combined usage -->
<style>
#box {
width: 100px;
height: 100px;
background: lightblue;
cursor: pointer;
}
</style>
<div id="box" onclick="this.style.background = 'lightgreen'">
Click to Change Color
</div>
HTML id Attribute Explained: Syntax, Usage & Best Practices
As a rule of thumb, you should always start id with a letter, avoid spaces, and use hyphens or underscores for better readability. For example, use id="header-section"
rather than id="1header"
. This approach aligns with both html id syntax and broader html unique identifier conventions.
Comprehensive HTML id Reference Table
This table consolidates essential facts about the HTML id
attribute, covering its syntax, usage as a css html id selector and in html id javascript, anchor linking, accessibility. You should always follow these practices so that you can implement unique identifiers with confidence.
id
<div id="intro">…</div>
section-1
<img id="logo">
#id
.#intro { color: blue; }
document.getElementById()
to select the element.document.getElementById('intro')
#id
in an anchor’s href.<a href="#intro">Go to Intro</a>
<section id="features">…</section>
id="Header"
≠ id="header"
(XHTML)user-profile_1.0
aria-labelledby
.<label id="lbl">Name</label>… aria-labelledby="lbl"
getElementById()
results.nav-main
footer-info
Web Resources on HTML id Attribute
1. University of South Carolina – “id Attribute” (concise rules, syntax, and examples)
2. University of Washington – Understanding ID in HTML
3. Stanford University – “When Working with Element IDs, Make Them Unique”
4. Stanford Sites User Guide — Guidance on in-page navigation via id anchors
5. MDN Web Docs “Global attributes: id” — syntax, examples, and browser compatibility
Questions and Answers related to HTML Id
In HTML, the id
attribute assigns a unique identifier to an element, ensuring no two elements share the same id
within a document. This uniqueness allows for precise targeting in CSS for styling and in JavaScript for DOM manipulation. For example, <div id="header"></div>
creates a div
element with the unique identifier “header”.
To style an element with a specific id
in CSS, use the #
symbol followed by the id
name. For instance, to style a div
with the id
“content”, define the CSS rule as #content { /* styles here */ }
. This targets the element with id="content"
for styling.
In HTML, the id
attribute provides a unique identifier for a single element, while the class
attribute can be assigned to multiple elements to apply shared styling or behavior. For example, multiple elements can have class="button"
to share the same styles, but each should have a unique id
for individual identification.
To create an anchor link to a specific section, assign an id
to the target element, e.g., <section id="about"></section>
. Then, create a hyperlink pointing to this id
with <a href="#about">About</a>
. Clicking the link will navigate to the section with id="about"
.
Best practices for id
naming include using descriptive, meaningful names that reflect the element’s purpose, starting with a letter, and avoiding spaces or special characters. For example, use id="mainHeader"
instead of id="h1"
to clearly indicate the element’s role.
In JavaScript, you can manipulate an element by its id
using document.getElementById("elementId")
. For example, to change the text of a paragraph with id="intro"
, use document.getElementById("intro").innerHTML = "New text";
. This targets the specific element for dynamic content updates.
HTML id
attributes must start with a letter (A-Z or a-z) and can be followed by letters, digits (0-9), hyphens (“-“), underscores (“_”), colons (“:”), and periods (“.”). They cannot start with a digit or contain spaces. Adhering to these rules ensures valid, accessible HTML.
To assign an id
to a div
element, include the id
attribute within the opening tag, such as <div id="uniqueId"></div>
. For example, <div id="mainContent"></div>
creates a div
with the id
“mainContent”. To combine id
and class
, you can write <div id="mainContent" class="content"></div>
, where “mainContent” is the unique identifier and “content” is a class that can be applied to multiple elements.
In HTML, class
and id
attributes are used to assign identifiers to elements for styling and scripting. The id
attribute provides a unique identifier for a single element, while the class
attribute can be assigned to multiple elements to apply shared styling or behavior. For example, multiple elements can have class="button"
to share the same styles, but each should have a unique id
for individual identification. To use them effectively, apply id
for unique elements and class
for groups of elements that share common styles or behaviors.
The id
attribute in HTML assigns a unique identifier to an element, ensuring no two elements share the same id
within a document. This uniqueness allows for precise targeting in CSS for styling and in JavaScript for DOM manipulation. For example, <div id="header"></div>
creates a div
element with the unique identifier “header”. This enables developers to apply specific styles or behaviors to that element without affecting others.
You can Edit the codes Here
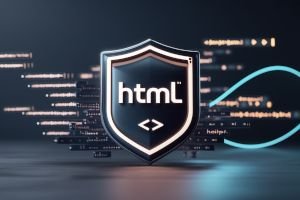
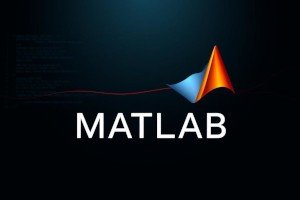
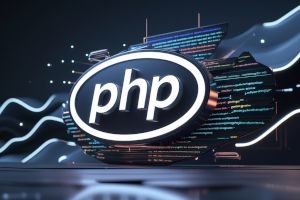
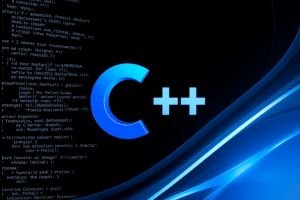