HTML Image Tag
Mastering the HTML Image Tag and Attributes
HTML images bring life to your webpages by embedding visuals directly into your content. In this webpage, we will explore how to use the <img>
tag, and optimize it with alt tags for images, add background image, add image border, align images, rotate an image, Overlay text over image and create image galleries. In addition you will also learn how to create clickable Areas on the Image, and create attractive image sliders.
Examples of HTML Images
1. Simple <img>
Tag with Alt Attribute
The simple <img> tag is used to display images in HTML, while the alt attribute provides alternative text for accessibility and SEO. You should include descriptive alt text to ensure that your content remains informative even if the image fails to load. It also provides information about your image to search engines.
<!-- Example: Basic HTML image tag -->
<!DOCTYPE html>
<html>
<head>
<title>Basic Image Example</title>
</head>
<body>
<h2>HTML Image Tag</h2>
<img src="https://www.academicblock.net/wp-content/uploads/2025/04/Bird-01-AB.jpg" alt="Image of a bird." width="250">
<p> Image of a beautiful bird. </p>
</body>
</html>
2. Add Background Image in your webpage
The style attribute can also be used to add a background image in HTML using background-image: url(‘image.jpg’). This inline method is useful for quick styling without external CSS, though it’s best used for simple layouts or testing purposes.
<!-- Example: Inline background image -->
<!DOCTYPE html>
<html>
<head>
<title>Background Image Example</title>
</head>
<body style="background-image: url('https://www.academicblock.net/wp-content/uploads/2025/05/Light-Background-AB.jpg'); background-size: cover;">
<h2>HTML Background Image</h2>
<p>This div uses inline CSS to set a background image.</p>
</body>
</html>
3. Add Border Around the Image
To emphasize an image, you can easily add a border using inline CSS on the img tag
.
This demonstrates the power of html image attributes and helps control your
HTML image size for a polished presentation.
<!-- Example: Adding a border around an image -->
<!DOCTYPE html>
<html>
<head>
<title>Image Border Example</title>
</head>
<body>
<h2>Border Around HTML Image</h2>
<img
src="https://www.academicblock.net/wp-content/uploads/2025/05/Lilttle-Baby-AB.jpg"
alt="Photo with border"
style="border: 5px solid red; width: 300px; height: auto;"
>
</body>
</html>
4. Align Image (Left, Right, Center) in a Webpage
You can align images in your layout using CSS. Float images to the left or right, or center them within a webpage. This technique enhances your html responsive images and page flow.
<!-- Example: Aligning images -->
<!DOCTYPE html>
<html>
<head>
<title>Image Alignment Example</title>
</head>
<body>
<h2>Align Images in HTML</h2>
<!-- Float left -->
<img
src="https://www.academicblock.net/wp-content/uploads/2025/05/Image-Left-AB.png"
alt="Left aligned"
style="float: left; margin-bottom: 100px; width: 75px;"
> <br> <br>
<!-- Float right -->
<img
src="https://www.academicblock.net/wp-content/uploads/2025/05/Image-Right-AB.png"
alt="Right aligned"
style="float: right; margin-top: 50px; margin-bottom: 50px; width: 75px;"
> <br> <br>
<!-- Center -->
<div style="text-align: center; clear: both; margin-top: 30px;">
<img
src="https://www.academicblock.net/wp-content/uploads/2025/05/Image-Center-AB.png"
alt="Centered image"
style="display: inline-block; width: 75px;"
>
</div>
</body>
</html>
5. Show Rotated Image (at Angle) in a Webpage
We can use CSS transforms to rotate an image to any angle. Example below showcases modern CSS images capabilities and creative control over your html image tag.
<!-- Example: Rotating an image -->
<!DOCTYPE html>
<html>
<head>
<title>Rotate Image Example</title>
</head>
<body>
<h2>Rotated HTML Image</h2>
<img
src="https://www.academicblock.net/wp-content/uploads/2025/05/Lilttle-Baby-AB.jpg"
alt="Rotated image"
style="transform: rotate(15deg); display: block; margin: 50px auto; width: 250px;"
>
</body>
</html>
6. Create Clickable Areas on the Image using HTML
We can define interactive zones on a single image using an HTML image map. This technique allows different parts of a single image to direct users to various destinations, enhancing interactivity and navigation.
Note:
1. coords=”35,145,100,190″: These are the pixel coordinates for the rectangle’s top-left corner (35, 145) and bottom-right corner (100, 190) of the input image. These define the clickable rectangular area on the image.
2. coords=”185,131,23″: These are the pixel coordinates for the center of the circle (185, 131) and the radius of the circle (23). This defines the clickable circular area on the image.
<!-- Example: Image map with clickable areas -->
<!DOCTYPE html>
<html>
<head>
<title>Image Map Example</title>
</head>
<body>
<h2>Clickable Areas on HTML Image</h2>
<img
src="https://www.academicblock.net/wp-content/uploads/2025/05/World-Map-01-AB-1.jpg"
alt="World map"
usemap="#worldmap"
style="width: 350px;"
>
<map name="worldmap">
<area shape="rect" coords="35,145,100,190" href="north-america.html" alt="North America">
<area shape="circle" coords="185,131,23" href="europe.html" alt="Europe">
</map>
</body>
</html>
7. Overlay Text on the Image
To put text over an image in HTML, you can use a container like a <div> with CSS to position the text on top of the image. This is great for adding titles, labels, or buttons directly on pictures, like in banners or ads.
<!-- Example: Text overlay on image -->
<!DOCTYPE html>
<html>
<head>
<title>Image Text Overlay</title>
</head>
<body>
<h2>Overlay Text on HTML Image</h2>
<div style="position: relative; display: inline-block;">
<img
src="https://www.academicblock.net/wp-content/uploads/2025/05/Smooth-Color-Gradient-1-rotated.jpg"
alt="Banner"
style="width: 500px;"
>
<div style="
position: absolute;
bottom: 20px;
left: 20px;
color: white;
background: rgba(0, 0, 0, 0.5);
padding: 5px 10px;
font-size: 1.2em;
">
Welcome to Our Site
</div>
</div>
</body>
</html>
8. Create Image Gallery Using Grid Box
You can create an image gallery using HTML and CSS Grid by placing images inside a container styled with display: grid. This layout helps arrange pictures in neat rows and columns, making your gallery clean, organized, and responsive. This can be also seen in the example below:
<!-- Example: CSS Grid image gallery -->
<!DOCTYPE html>
<html>
<head>
<title>Grid Image Gallery</title>
</head>
<body>
<h2>HTML Image Gallery with Grid</h2>
<div style="
display: grid;
grid-template-columns: repeat(auto-fit, minmax(150px, 1fr));
gap: 10px;
">
<img src="https://www.academicblock.net/wp-content/uploads/2025/05/Tiger-AB.jpg" alt="Grid image 1" style="width: 90%; height: auto;">
<img src="https://www.academicblock.net/wp-content/uploads/2025/05/Elephant-AB.jpg" alt="Grid image 2" style="width: 90%; height: auto;">
<img src="https://www.academicblock.net/wp-content/uploads/2025/05/Bear-AB.jpg" alt="Grid image 3" style="width: 90%; height: auto;">
<img src="https://www.academicblock.net/wp-content/uploads/2025/05/Rabbit-AB.jpg" alt="Grid image 4" style="width: 90%; height: auto;">
</div>
</body>
</html>
9. Create Simple Manual Image Slider
A simple manual image slider can be built using HTML, CSS, and a little JavaScript, where images are changed when buttons are clicked. A simple example of this given below:
<!-- Example: Manual image slider -->
<!DOCTYPE html>
<html>
<head>
<title>Manual Image Slider</title>
</head>
<body>
<h2 style="text-align: center;" >Simple Manual Slider</h2>
<div style="text-align: center;">
<button onclick="prev()">◀ Prev</button>
<img
id="slider"
src="https://www.academicblock.net/wp-content/uploads/2025/05/Tiger-AB.jpg"
alt="Slide 1"
style="width: 150px; height: auto; vertical-align: middle;"
>
<button onclick="next()">Next ▶</button>
</div>
<script>
const slides = ['https://www.academicblock.net/wp-content/uploads/2025/05/Tiger-AB.jpg','https://www.academicblock.net/wp-content/uploads/2025/05/Elephant-AB.jpg','https://www.academicblock.net/wp-content/uploads/2025/05/Bear-AB.jpg'];
let idx = 0;
function next() {
idx = (idx + 1) % slides.length;
document.getElementById('slider').src = slides[idx];
document.getElementById('slider').alt = 'Slide ' + (idx + 1);
}
function prev() {
idx = (idx - 1 + slides.length) % slides.length;
document.getElementById('slider').src = slides[idx];
document.getElementById('slider').alt = 'Slide ' + (idx + 1);
}
</script>
</body>
</html>
<img> Tag Attributes (Expanded)
The table below lists the most common image attributes, it explains what each one does, and gives a real-world example. Using these attributes—like src, alt, loading, and width—helps your images load faster, look better on different devices, and is accessible to everyone.
Web Resources on HTML Images
1. CSUN: HTML Tutorial – Images
2. Colorado State University: HTML Image Tags
3. University of Rochester: HTML Tutorial (Images)
4. Duke University: HTML Tutorial –Images
5. MDN Web Docs: HTML images
Questions and Answers related to HTML Image Tag and Attributes
HTML images are added using the <img>
tag. The src
attribute specifies the image source, which can be a URL or a local file. Example: <img src="image.jpg" alt="Description">
. Always include an alt
attribute for accessibility and SEO benefits.
The alt
attribute provides alternative text for images, enhancing accessibility for visually impaired users and improving SEO. Example: <img src="logo.png" alt="Company Logo">
. If an image fails to load, the alt text is displayed instead.
To add an image from a folder, use: <img src="images/pic.jpg" alt="Sample Image">
. Ensure the file path is correct. Place your HTML file in the same directory or provide a relative path for proper embedding.
Background images are added using CSS: background-image: url('bg.jpg');
. Apply it to an element like <body>
or a <div>
. Use background-size: cover;
for full coverage.
Responsive images adjust to different screen sizes. Use the srcset
attribute: <img src="default.jpg" srcset="small.jpg 600w, large.jpg 1200w">
. Optimize using compression and WebP format.
Use a <div>
container with <img>
tags. Example: <div class="gallery"><img src="pic1.jpg"><img src="pic2.jpg"></div>
. Style using CSS grid or flexbox.
Add a favicon using <link rel="icon" href="favicon.ico">
. A banner is an <img>
element, while a background image is applied via CSS.
Wrap the <img>
tag inside an <a>
tag: <a href="page.html"><img src="image.jpg"></a>
. Clicking the image navigates to the link.
Use <img>
for content images and CSS background-image
for decorative images. Content images need alt
attributes for SEO.
Set size using width
and height
attributes: <img src="pic.jpg" width="300" height="200">
. Use CSS for responsive sizing.
You can Edit the codes Here
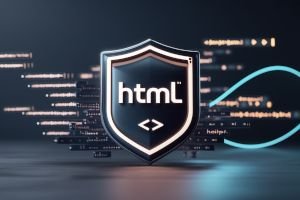
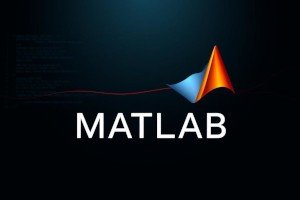
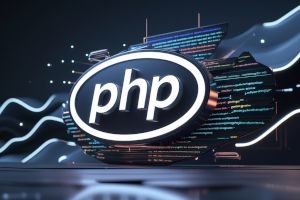
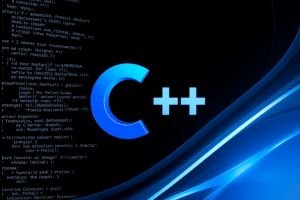